//SearchIPAdress 类(SearchIPAdress.cs)
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
namespace QQClient
{
public partial class SearchIPAdress : Form
{
public SearchIPAdress()
{
InitializeComponent();
initData();
}
void initData() {
string outIP = ClassIPSearch.getOutIP();
textBoxInnerIP.Text = ClassIPSearch.GetInnerIP();
textBoxOutIP.Text = outIP;
string location = ClassIPSearch.getOutLocation(outIP);
labelLocation.Text = location;
}
private void buttonSearch1_Click(object sender, EventArgs e)
{
textBoxOutIP.Text = ClassIPSearch.getOutIP();
}
private void buttonSearch2_Click(object sender, EventArgs e)
{
string outIP2 = textBoxOutIP2.Text.ToString();
if (outIP2 == "" || outIP2 == null) {
outIP2 = textBoxInnerIP2.Text.ToString();
}
string location2 = ClassIPSearch.getOutLocation(outIP2);
labelLocation2.Text = location2;
if (outIP2.Equals(textBoxOutIP.Text.ToString()) || location2.Equals("本地局域网"))
textBoxYN.Text = "是";
else
textBoxYN.Text = "不是";
}
}
}
//ClassIPSearch类
using System;
using System.Collections.Generic;
using System.Web;
using System.Text.RegularExpressions;
using System.Collections;
using System.Net;
using System.Windows.Forms;
using System.Text;
namespace QQClient
{
public class ClassIPSearch {
// 获取外网IP
public static string getOutIP()
{
string strUrl = "http://www.ip138.com/ip2city.asp"; //获得IP的网址了
Uri uri = new Uri(strUrl);
System.Net.WebRequest wr = System.Net.WebRequest.Create(uri);
System.IO.Stream s = wr.GetResponse().GetResponseStream();
System.IO.StreamReader sr = new System.IO.StreamReader(s, Encoding.Default);
string all = sr.ReadToEnd(); //读取网站的数据
int i = all.IndexOf("[") + 1;
string tempip = all.Substring(i, 15);
string ip = tempip.Replace("]", "").Replace(" ", "");//找出i
return ip;
}
// 获取本机的地理位置
public static string getOutLocation(string ipAddress)
{
string m_Location = "", m_IpAddress = "", m_Response = "";
m_IpAddress = ipAddress.Trim();
WebClient client = new WebClient();
client.Encoding = System.Text.Encoding.GetEncoding("GB2312");
string url = "http://www.ip138.com/ips.asp";
string post = "ip=" + ipAddress + "&action=2";
client.Headers.Set("Content-Type", "application/x-www-form-urlencoded");
string response = client.UploadString(url, post);
m_Response = response;
string p = @"<li>参考数据一:(?<location>[^<>]+?)</li>";
Match match = Regex.Match(response, p);
m_Location = match.Groups["location"].Value.Trim();
return m_Location;
}
//本机局域网IP
public static string GetInnerIP()
{
IPHostEntry ipEntry = Dns.GetHostEntry(Dns.GetHostName()); //取得本机IP
return ipEntry.AddressList[0].ToString();
}
}
}
//设计代码(SearchIPAdress.Designer.cs)
namespace QQClient
{
partial class SearchIPAdress
{
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.IContainer components = null;
/// <summary>
/// Clean up any resources being used.
/// </summary>
/// <param name="disposing">true if managed resources should be disposed; otherwise, false.</param>
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.textBoxInnerIP = new System.Windows.Forms.TextBox();
this.label1 = new System.Windows.Forms.Label();
this.label2 = new System.Windows.Forms.Label();
this.buttonSearch2 = new System.Windows.Forms.Button();
this.label3 = new System.Windows.Forms.Label();
this.textBoxOutIP = new System.Windows.Forms.TextBox();
this.textBoxOutIP2 = new System.Windows.Forms.TextBox();
this.label4 = new System.Windows.Forms.Label();
this.label5 = new System.Windows.Forms.Label();
this.textBoxInnerIP2 = new System.Windows.Forms.TextBox();
this.buttonSearch1 = new System.Windows.Forms.Button();
this.label6 = new System.Windows.Forms.Label();
this.labelLocation = new System.Windows.Forms.Label();
this.labelLocation2 = new System.Windows.Forms.Label();
this.label8 = new System.Windows.Forms.Label();
this.label10 = new System.Windows.Forms.Label();
this.label11 = new System.Windows.Forms.Label();
this.textBoxYN = new System.Windows.Forms.TextBox();
this.SuspendLayout();
//
// textBoxInnerIP
//
this.textBoxInnerIP.BackColor = System.Drawing.Color.White;
this.textBoxInnerIP.Font = new System.Drawing.Font("宋体", 13F);
this.textBoxInnerIP.Location = new System.Drawing.Point(232, 74);
this.textBoxInnerIP.MaxLength = 15;
this.textBoxInnerIP.Name = "textBoxInnerIP";
this.textBoxInnerIP.ReadOnly = true;
this.textBoxInnerIP.Size = new System.Drawing.Size(162, 27);
this.textBoxInnerIP.TabIndex = 0;
//
// label1
//
this.label1.AutoSize = true;
this.label1.Font = new System.Drawing.Font("宋体", 21.75F, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, ((byte)(134)));
this.label1.Location = new System.Drawing.Point(88, 19);
this.label1.Name = "label1";
this.label1.Size = new System.Drawing.Size(315, 29);
this.label1.TabIndex = 1;
this.label1.Text = "搜索IP地址的地理位置";
//
// label2
//
this.label2.AutoSize = true;
this.label2.Font = new System.Drawing.Font("宋体", 14.25F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((byte)(134)));
this.label2.Location = new System.Drawing.Point(54, 79);
this.label2.Name = "label2";
this.label2.Size = new System.Drawing.Size(172, 19);
this.label2.TabIndex = 2;
this.label2.Text = "您的内网IP地址是:";
//
// buttonSearch2
//
this.buttonSearch2.Font = new System.Drawing.Font("宋体", 14.25F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((byte)(134)));
this.buttonSearch2.Location = new System.Drawing.Point(411, 241);
this.buttonSearch2.Name = "buttonSearch2";
this.buttonSearch2.Size = new System.Drawing.Size(75, 29);
this.buttonSearch2.TabIndex = 7;
this.buttonSearch2.Text = "查询";
this.buttonSearch2.UseVisualStyleBackColor = true;
this.buttonSearch2.Click += new System.EventHandler(this.buttonSearch2_Click);
//
// label3
//
this.label3.AutoSize = true;
this.label3.Font = new System.Drawing.Font("宋体", 14.25F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((byte)(134)));
this.label3.Location = new System.Drawing.Point(54, 119);
this.label3.Name = "label3";
this.label3.Size = new System.Drawing.Size(172, 19);
this.label3.TabIndex = 10;
this.label3.Text = "您的外网IP地址是:";
//
// textBoxOutIP
//
this.textBoxOutIP.BackColor = System.Drawing.Color.White;
this.textBoxOutIP.Font = new System.Drawing.Font("宋体", 13F);
this.textBoxOutIP.Location = new System.Drawing.Point(232, 112);
this.textBoxOutIP.MaxLength = 15;
this.textBoxOutIP.Name = "textBoxOutIP";
this.textBoxOutIP.ReadOnly = true;
this.textBoxOutIP.Size = new System.Drawing.Size(162, 27);
this.textBoxOutIP.TabIndex = 11;
//
// textBoxOutIP2
//
this.textBoxOutIP2.BackColor = System.Drawing.Color.White;
this.textBoxOutIP2.Font = new System.Drawing.Font("宋体", 13F);
this.textBoxOutIP2.ForeColor = System.Drawing.SystemColors.WindowText;
this.textBoxOutIP2.Location = new System.Drawing.Point(238, 242);
this.textBoxOutIP2.MaxLength = 15;
this.textBoxOutIP2.Name = "textBoxOutIP2";
this.textBoxOutIP2.Size = new System.Drawing.Size(162, 27);
this.textBoxOutIP2.TabIndex = 15;
this.textBoxOutIP2.TextAlign = System.Windows.Forms.HorizontalAlignment.Center;
//
// label4
//
this.label4.AutoSize = true;
this.label4.Font = new System.Drawing.Font("宋体", 14.25F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((byte)(134)));
this.label4.Location = new System.Drawing.Point(59, 244);
this.label4.Name = "label4";
this.label4.Size = new System.Drawing.Size(172, 19);
this.label4.TabIndex = 14;
this.label4.Text = "他的外网IP地址是:";
//
// label5
//
this.label5.AutoSize = true;
this.label5.Font = new System.Drawing.Font("宋体", 14.25F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((byte)(134)));
this.label5.Location = new System.Drawing.Point(58, 204);
this.label5.Name = "label5";
this.label5.Size = new System.Drawing.Size(172, 19);
this.label5.TabIndex = 13;
this.label5.Text = "他的内网IP地址是:";
//
// textBoxInnerIP2
//
this.textBoxInnerIP2.BackColor = System.Drawing.Color.White;
this.textBoxInnerIP2.Font = new System.Drawing.Font("宋体", 13F);
this.textBoxInnerIP2.ForeColor = System.Drawing.SystemColors.WindowText;
this.textBoxInnerIP2.Location = new System.Drawing.Point(238, 202);
this.textBoxInnerIP2.MaxLength = 15;
this.textBoxInnerIP2.Name = "textBoxInnerIP2";
this.textBoxInnerIP2.Size = new System.Drawing.Size(162, 27);
this.textBoxInnerIP2.TabIndex = 12;
this.textBoxInnerIP2.TextAlign = System.Windows.Forms.HorizontalAlignment.Center;
//
// buttonSearch1
//
this.buttonSearch1.Enabled = false;
this.buttonSearch1.Font = new System.Drawing.Font("宋体", 14.25F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((byte)(134)));
this.buttonSearch1.Location = new System.Drawing.Point(410, 73);
this.buttonSearch1.Name = "buttonSearch1";
this.buttonSearch1.Size = new System.Drawing.Size(75, 29);
this.buttonSearch1.TabIndex = 16;
this.buttonSearch1.Text = "查询";
this.buttonSearch1.UseVisualStyleBackColor = true;
this.buttonSearch1.Click += new System.EventHandler(this.buttonSearch1_Click);
//
// label6
//
this.label6.AutoSize = true;
this.label6.Font = new System.Drawing.Font("宋体", 14.25F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((byte)(134)));
this.label6.Location = new System.Drawing.Point(75, 159);
this.label6.Name = "label6";
this.label6.Size = new System.Drawing.Size(152, 19);
this.label6.TabIndex = 17;
this.label6.Text = "您的地理位置是:";
//
// labelLocation
//
this.labelLocation.AutoSize = true;
this.labelLocation.Font = new System.Drawing.Font("宋体", 14F);
this.labelLocation.Location = new System.Drawing.Point(228, 159);
this.labelLocation.Name = "labelLocation";
this.labelLocation.Size = new System.Drawing.Size(85, 19);
this.labelLocation.TabIndex = 18;
this.labelLocation.Text = "地理位置";
//
// labelLocation2
//
this.labelLocation2.AutoSize = true;
this.labelLocation2.Font = new System.Drawing.Font("宋体", 14F);
this.labelLocation2.Location = new System.Drawing.Point(235, 283);
this.labelLocation2.Name = "labelLocation2";
this.labelLocation2.Size = new System.Drawing.Size(85, 19);
this.labelLocation2.TabIndex = 20;
this.labelLocation2.Text = "地理位置";
//
// label8
//
this.label8.AutoSize = true;
this.label8.Font = new System.Drawing.Font("宋体", 14.25F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((byte)(134)));
this.label8.Location = new System.Drawing.Point(82, 283);
this.label8.Name = "label8";
this.label8.Size = new System.Drawing.Size(152, 19);
this.label8.TabIndex = 19;
this.label8.Text = "您的地理位置是:";
//
// label10
//
this.label10.AutoSize = true;
this.label10.Font = new System.Drawing.Font("宋体", 14.25F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((byte)(134)));
this.label10.Location = new System.Drawing.Point(160, 316);
this.label10.Name = "label10";
this.label10.Size = new System.Drawing.Size(66, 19);
this.label10.TabIndex = 21;
this.label10.Text = "他与您";
//
// label11
//
this.label11.AutoSize = true;
this.label11.Font = new System.Drawing.Font("宋体", 14F);
this.label11.Location = new System.Drawing.Point(329, 317);
this.label11.Name = "label11";
this.label11.Size = new System.Drawing.Size(180, 19);
this.label11.TabIndex = 23;
this.label11.Text = "在同一个局域网内。";
//
// textBoxYN
//
this.textBoxYN.Font = new System.Drawing.Font("宋体", 14F);
this.textBoxYN.Location = new System.Drawing.Point(238, 314);
this.textBoxYN.Multiline = true;
this.textBoxYN.Name = "textBoxYN";
this.textBoxYN.Size = new System.Drawing.Size(82, 27);
this.textBoxYN.TabIndex = 24;
this.textBoxYN.Text = "是/不是";
this.textBoxYN.TextAlign = System.Windows.Forms.HorizontalAlignment.Center;
//
// SearchIPAdress
//
this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 12F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(569, 355);
this.Controls.Add(this.textBoxYN);
this.Controls.Add(this.label11);
this.Controls.Add(this.label10);
this.Controls.Add(this.labelLocation2);
this.Controls.Add(this.label8);
this.Controls.Add(this.labelLocation);
this.Controls.Add(this.label6);
this.Controls.Add(this.buttonSearch1);
this.Controls.Add(this.textBoxOutIP2);
this.Controls.Add(this.label4);
this.Controls.Add(this.label5);
this.Controls.Add(this.textBoxInnerIP2);
this.Controls.Add(this.textBoxOutIP);
this.Controls.Add(this.label3);
this.Controls.Add(this.buttonSearch2);
this.Controls.Add(this.label2);
this.Controls.Add(this.label1);
this.Controls.Add(this.textBoxInnerIP);
this.MinimumSize = new System.Drawing.Size(512, 332);
this.Name = "SearchIPAdress";
this.Text = "AddIPAdress";
this.ResumeLayout(false);
this.PerformLayout();
}
#endregion
private System.Windows.Forms.Label label1;
private System.Windows.Forms.Label label2;
private System.Windows.Forms.Button buttonSearch2;
public System.Windows.Forms.TextBox textBoxInnerIP;
private System.Windows.Forms.Label label3;
public System.Windows.Forms.TextBox textBoxOutIP;
public System.Windows.Forms.TextBox textBoxOutIP2;
private System.Windows.Forms.Label label4;
private System.Windows.Forms.Label label5;
public System.Windows.Forms.TextBox textBoxInnerIP2;
private System.Windows.Forms.Button buttonSearch1;
private System.Windows.Forms.Label label6;
private System.Windows.Forms.Label labelLocation;
private System.Windows.Forms.Label labelLocation2;
private System.Windows.Forms.Label label8;
private System.Windows.Forms.Label label10;
private System.Windows.Forms.Label label11;
private System.Windows.Forms.TextBox textBoxYN;
}
}
效果图:
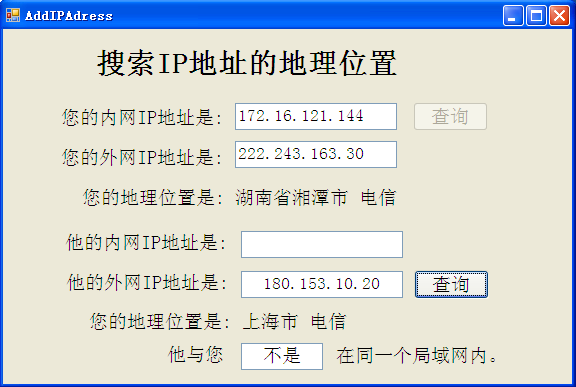
分享到:
相关推荐
C#通过IP地址获取主机名称
C#实现IP地址查询和手机号码归属地查询,是个不错的例子,需要的同学可以下载来看看
C#检测IP地址是否合法
C# 设置ip地址 vs 可以作为学习设置ip的小程序
可以设置和读取IP地址,具有跟Window的IP地址输入框一样的功能,箭头方向键、空格、句点、删除键可以在四个IP地址段内连续移动,可拉伸控件,改变字体颜色及大小,改变背景颜色及IP地址框的背景颜色。对输入的IP地址...
类似windowsIp地址输入框的自定义控件
使用正则表达式来判断用户输入的IP地址格式是否正确
C# 判断IP地址是否能够PING通
通过c#语言功能,修改电脑的IP地址及DNS.源码。
新学习 C# 程序 利用 MaskedTextBox 实现 IP 地址输入的判断例子!
本程序可以手动设置IP 地址等参数也可以自动获取IP地址的功能,希望对大家有所帮助
C#实现获取多网卡IP地址方法,该方法可以遍历出所有网卡的IP地址。
C#操作IP地址数据库源码(完整版)
C#通过IP地址查询google地图的坐标C#通过IP地址查询google地图的坐标
C#IP地址切换,可添加多个备用地址以备切换。支持多网卡,支持Win7、XP系统。环境需要:.Net 2.0环境。
运用C#编写检查可用的Ip地址,运用正则表达式验证Ip的有效性...
C# IP库地址查询 C# IP库地址查询
C# IP地址切换源码 实现备份网络配置切换,源码提供,实用方便。
根据网络上其它的经验 本人写的一个ip地址输入校验框 希望大家下载试用一下 有什么问题 可以提出来
C# 获取本地IP地址以及MAC地址C# 获取本地IP地址以及MAC地址